PyCon Italia 2019
Internet delle cose con Redis e django-channels
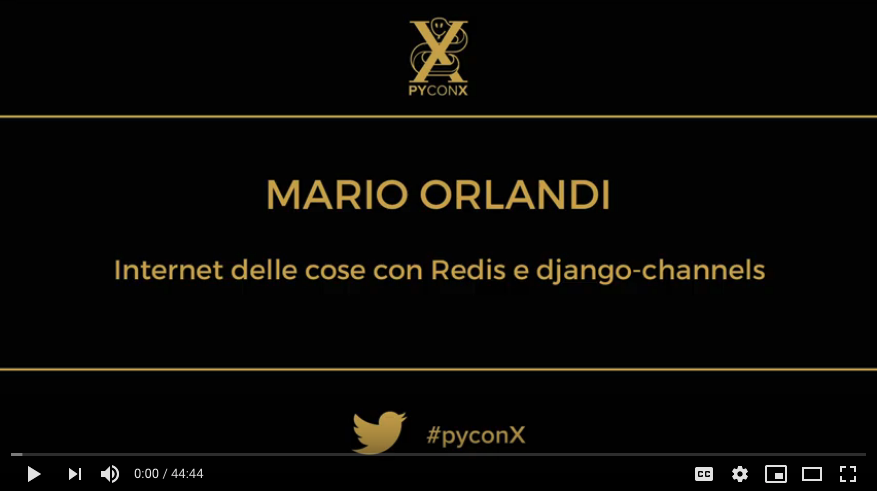
Development of vertical software solutions for medium to large businesses
Intranet, extranet, web services and IOT
Focused on technologies such as Python, Django, Redis, WebSockets, HTMX, IoT.
Real-time data acquisition, from Arduino to the web, using PubSub with Redis, Django and other friends
A Django app which provides advanced integration for a Django project with the jQuery Javascript library DataTables.net, when used in server-side processing mode.
Demo site
A Django helper app to add editing capabilities to the frontend using modal forms.
Demo site
A Django app to run new background tasks from either admin or cron, and inspect task history from admin; based on django-rq
A collection of tools to trace, analyze and render Querysets
Helper used to remove oldest records from specific db tables in a Django project
Realizzato allo scopo di verificare il codice utilizzato internamente nelle nostre applicazioni per l'accesso ai databases mediante l'interfaccia ADO, consente di eseguire query interattive su un generico database (MySQL, Access, SQL Server, Oracle, ecc...). E' inoltre possibile esportare la struttura e il contentuto di un generico database in forma di script SQL compatibile con MySQL oppure SQL Server.
Click here to downloadConsente di inviare dati ASCII oppure binari ad un generico dispositivo, sia utilizzando la comunicazione seriale, che via ethernet (TCP). E' inoltre possibile impostare risposte automatiche per simulare un generico protocollo di comunicazione. Supporta connessioni seriali e/o Ethernet, TCP o UDP (sia lato client che lato server), HTTP, e il protocollo seriale XModem.
Click here to downloadUtilizzato per la distribuzione dei sorgenti in forma crittata. La chiave di decodifica viene fornita singolarmente a ciascun cliente.
Click here to downloadBRAINSTORM S.r.l. di Mario Orlandi & C.
Viale Crispi, 2
41121 Modena, Italy
P.IVA 02409140361
Codice SDI: USAL8PV
Indirizzo Pec: brainstormsnc@pec.it
Tel: (+39) 059 216138
Insert your credentials to access protected services